Convert numbers to words currency (pt-pt, pt-br, en-us) the confusion!!
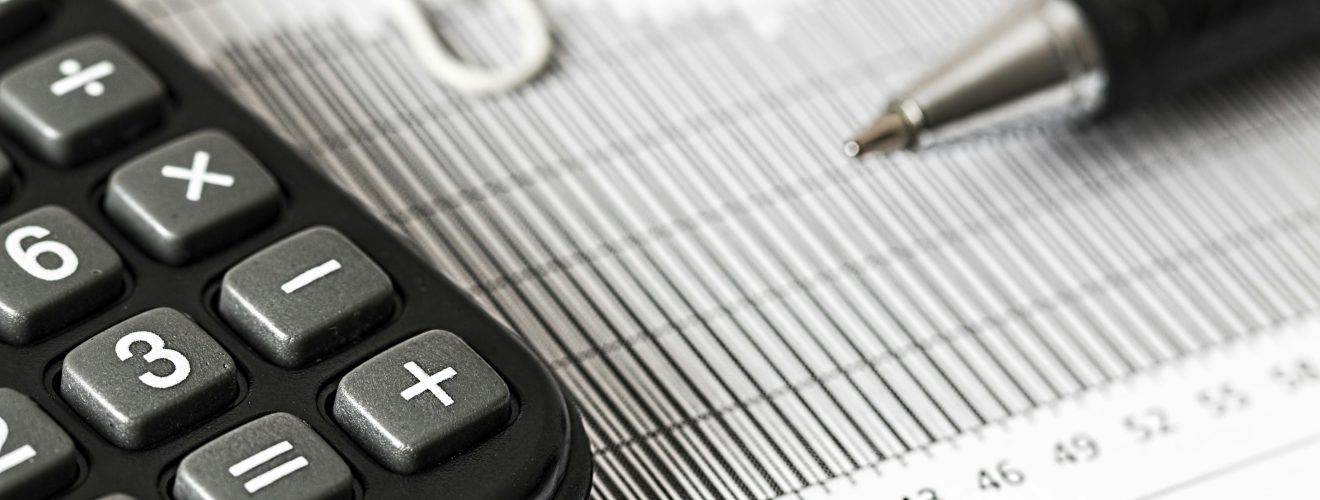
This is a know issue for converting numbers to words (Currency). There is some confusion in this concept, because not all countries use the same standard. Portugal uses the same nomenclature as most European countries, such as Spain, Italy, France, England, Germany, etc. But for example, the United States and Brazil use another form to designate numbers, which sometimes generates some confusion.
Look at the table below
In English
1,000 = a thousand
1,000,000 = a million
1,000,000,000 = a billion
1,000,000,000,000 = a trillion
In Portuguese Brazil:
1.000 = um milhar
1.000.000 = um milhão
1.000.000.000 = um bilhão
1.000.000.000.000 = um trilião
In Portuguese Portugal:
1.000 = um milhar
1.000.000 = um milhão
1.000.000.000 = um milhar de milhões
1.000.000.000.000 = um bilião
The Portuguese Standard NP-18 of 1960 refers to the nomenclature of large numbers. This standard was officially adopted in Portugal by Ordinances No. 14 608 and 17 052, respectively on November 11, 1953, and March 4, 1959.
According to rule N of NP-18, the names of large numbers are obtained using the designatory expression 10^6N = (N)illion, where the successive values of N (2, 3, 4, 5, 6, etc.) are replaced by the Latin designations bi, tri, quatri, quinti, sexti, septi, octi, etc. For example, the number 1 000 000 000 000 = 10^12 = 10^6×2 is called a “bilião”.
CODE IS NOT COMPLETE, it’s only a sample to illustrate the article, thanks
// Function to convert numbers into currencies
function numberToCurrency(num: string, language: string): string {
// Replace comma with dot for decimal separator
num = num.replace(',', '.');
// Convert string to number
let number = parseFloat(num);
// Dictionaries for words in different languages
const words = {
'en': [
'zero',
'one',
'two',
'three',
'four',
'five',
'six',
'seven',
'eight',
'nine',
'ten',
'eleven',
'twelve',
'thirteen',
'fourteen',
'fifteen',
'sixteen',
'seventeen',
'eighteen',
'nineteen',
'twenty',
'thirty',
'forty',
'fifty',
'sixty',
'seventy',
'eighty',
'ninety',
'hundred',
'thousand',
'million',
'billion',
'trillion',
'quadrillion',
'quintillion',
'sextillion',
'septillion',
'octillion',
'nonillion',
'decillion'
],
'pt-br': [
'zero',
'um',
'dois',
'três',
'quatro',
'cinco',
'seis',
'sete',
'oito',
'nove',
'dez',
'onze',
'doze',
'treze',
'quatorze',
'quinze',
'dezesseis',
'dezessete',
'dezoito',
'dezenove',
'vinte',
'trinta',
'quarenta',
'cinquenta',
'sessenta',
'setenta',
'oitenta',
'noventa',
'cem',
'mil',
'milhão',
'bilhão',
'trilhão',
'quadrilhão',
'quintilhão',
'sextilhão',
'setilhão',
'octilhão',
'nonilhão',
'decilhão'
],
'pt-pt': [
'zero',
'um',
'dois',
'três',
'quatro',
'cinco',
'seis',
'sete',
'oito',
'nove',
'dez',
'onze',
'doze',
'treze',
'catorze',
'quinze',
'dezasseis',
'dezassete',
'dezoito',
'dezanove',
'vinte',
'trinta',
'quarenta',
'cinquenta',
'sessenta',
'setenta',
'oitenta',
'noventa',
'cem',
'mil',
'milhão',
'mil milhões',
'bilião',
'mil biliões',
'trilião',
'mil triliões',
'quadrilião',
'mil quadriliões',
'quintilião',
'mil quintiliões'
]
};
let result = '';
let i = 0;
let isNegative = false;
// Check if the number is negative
if (number < 0) {
isNegative = true;
number = Math.abs(number);
}
// Separate the integer and decimal part of the number
let integerPart = Math.floor(number);
let decimalPart = number - integerPart;
// Loop to convert each part of the number into words
while (integerPart > 0) {
if (integerPart % 1000 !== 0){
result = integerPart % 1000 + ' ' + words[language][i] + ' ' + result;
}
integerPart = Math.floor(integerPart / 1000);
i++;
}
// If the result is empty, it means the number is zero
if (result === '') {
result = words[language][0];
}
// Add the currency to the result
let currency = '';
if (language === 'en') {
currency = ' dollars';
} else if (language === 'pt-br') {
currency = ' reais';
} else if (language === 'pt-pt') {
currency = ' euros';
}
result += currency;
// Add the decimal part if it exists
if (decimalPart > 0) {
let centName = '';
if (language === 'en') {
centName = ' cents';
} else {
centName = ' cêntimos';
}
result += ' and ' + Math.round(decimalPart * 100) + centName;
}
// Add the negative sign if necessary
if (isNegative) {
result = '-' + result;
}
return result.trim();
}
// Testing the function with some numbers
console.log(numberToCurrency('0,50', 'en')); // Outputs: "zero dollars and 50 cents"
console.log(numberToCurrency('-0,99', 'pt-br')); // Outputs: "-zero reais e 99 cêntimos"
console.log(numberToCurrency('0,01', 'pt-pt')); // Outputs: "zero euros e 1 cêntimo"
In summary Mathematics is universal, but mathematical language is not. In Portugal, I use the word “quadrado” to designate a quadrilateral with 4 equal sides and angles, in England they use the word “square”. Although both designate the same, the word used is different. The same happens with numbers, the term used to designate a certain number changes according to the country of origin.