PnPJs SPFx orderBy() not working?
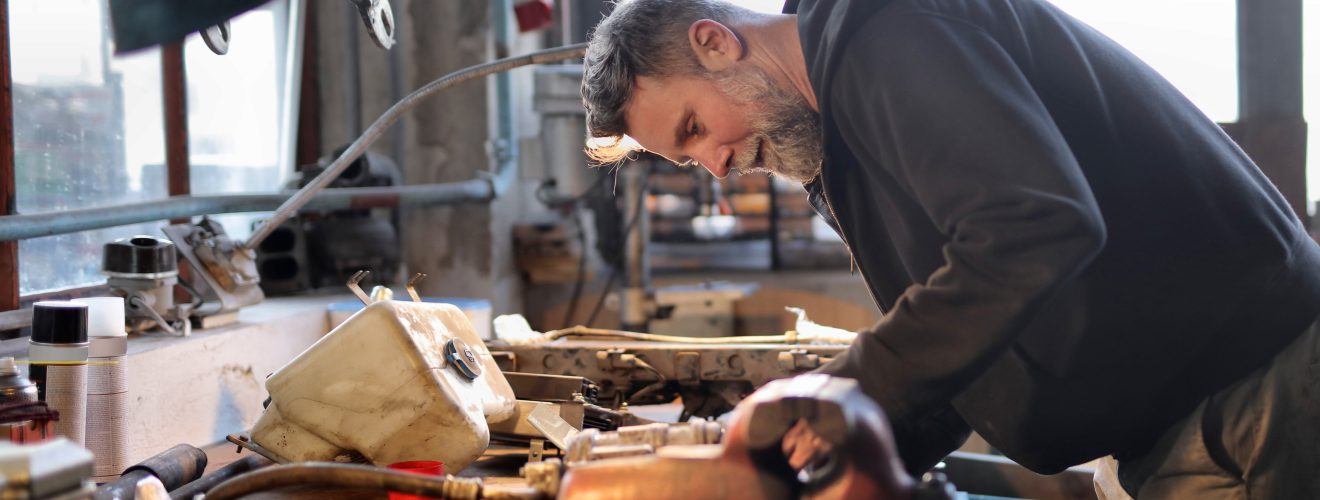
In the PnPJs Agnostic Framework Some fields are not sortable, let’s assume this call is using a MODEL where TrainingAreaPosition
is a number column without decimal places:
const [trainingPages, setPages] = useState<ISomeModel[]>([]);
//* Omitted for abbreviation */
const spCache = spfi(_sp).using(Caching({ store: "session" }))
const sortField: string = "TrainingAreaPosition";
const sortAsc: boolean = true;
const response: ISomeModelResponse[] = await spCache.web.lists
.getByTitle(LIBRARY_NAME)
.items.select("Id", "Title", "TrainingArea", "TrainingPageActive", "TrainingAreaPosition", "FileLeafRef")
.filter("TrainingPageActive eq 1")
.orderBy(sortField, sortAsc)
();
const items: ISomeModel[] = response.map((item: ISomeModelResponse) => {
return {
Id: item.Id,
Title: item.Title,
TrainingArea: item.TrainingArea,
Active: item.TrainingPageActive,
Position: item.TrainingAreaPosition,
Name: item.FileLeafRef
};
});
I’m using a Custom React Hook, fix by comparing the field name, where Position
is a property of the Model to Map
const byPosition = items.slice(0);
byPosition.sort(function (a, b) {
return a.Position - b.Position;
});
setPages(byPosition);
//* Omitted for abbreviation */
return [trainingPages, isError] as const
};
export default useTrainingPages